Trimble Installation Manager
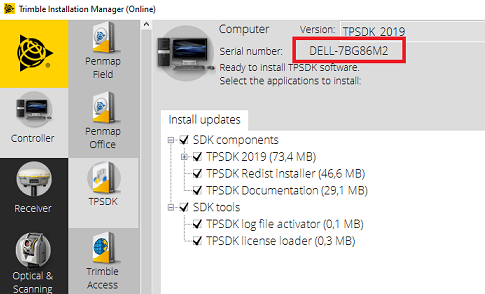
TPSDK is provided via Trimble Installation Manager (TIM) if the PC is licensed.
If you do not have a valid developer license you won’t see TPSDK in TIM.
To request/ renew a license please download and run TIM. In a first step it will display the serial. In a second step it will show TPSDK install options.
Once TIM is running copy/ paste the serial to an email and send an inquiry.
If you have no email client set up you can also contact TPSDK admin directly.
Sample applications
After installing the SDK you are ready to go.
The best starting point is the SSI sample application - which is a 1:1 map of all interfaces within TPSDK.
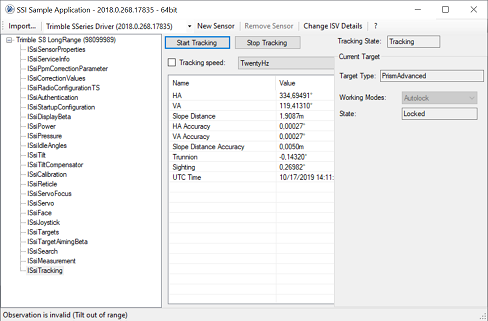
Each instrument type (or platform) has a defines feature set - which gets represented via interfaces. If you have an entry-level instrument you might only get a handful of supported interfaces while a high-end instruments supports a lot more.
Typical generic interfaces are e.g.
- ISsiPower
- ISsiSensorProperties or
- ISsiServiceInfo
- …
instrument class specific interfaces (total station in this case) are e.g.
- ISsiTargets
- ISsiMeasurement
- ISsiSearch
- …
The sample application should give you a rough idea on how the SDK works.
Note that interface availabilty is determined at runtime - there is no hardcoded list of supported interfaces. Hence a list of supported interfaces is created at runtime after a connection is made.
Next to the samples the documentation also covers a wide range of topics. Additionally to the default class documentation there is also a large text section which talks about measurement reduction (antenna phase center, prism, ppm etc.), the contract of licensing in general.
First solution
Creating a first solution in Microsoft® Visual Studio® which implements TPSDK is straight forward:
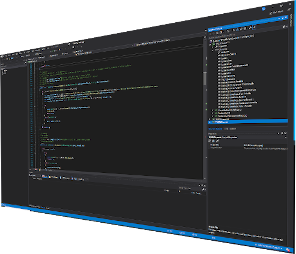
Create
Create a new .NET 4.8 (say C#) solution type.
Add
Add the required references and files to your solution. Note that TPSDK is a mix of managed and unmanaged code. Therefore you not only need to add references but also copy files/ keep a certain file structure.
References
Usually you add all interface dlls as references to your project. The interface dlls are located in two folder:
- \Bin*.dll
- \Windows\Bin*.dll
For simplicity just create a libs
folder in your solution and copy all dlls into this location (say libs\ssi\bin
for a very granular structure).
A none-comprehensive list looks like this:
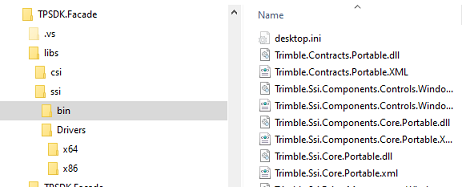
- Trimble.Contracts
- Trimble.Ssi.Components.Core
- Trimble.Ssi.Core
- Trimble.Ssi.Interfaces
- Trimble.Ssi.DriverManagement.Windows
- Trimble.Ssi.Interfaces.Gnss
- Trimble.Ssi.Interfaces.TotalStation
- Trimble.Ssi.Interfaces.Scanner
- Trimble.Ssi.Interfaces.Vision
- Trimble.Licensing.Windows
- LicensingV2Win
- LicensingV2WinCLRBridge
Files
Next we need to copy some native files - the drivers.
For that purpose a drivers
folders can be created next to the bin
folder; into this folder copy all files from the \Windows\Drivers*.* location.
Its required to keep the existing folder structure inside the drivers
. So the x64
and x86
must stay as sub folders in drivers
as the driver which gets loaded is actually proxy drivers linking to their native implementation (depending on the driver).
Create
Connecting to an instrument always means creating a driver manager - which is the container for all following drivers and sensors.
An application has to load the driver corresponding to the sensor the user wants to control. So for controlling a Trimble R12 receiver the
Trimble.Ssi.Driver.CarpoBased.Driver.RSeries.Windows.dll
must be loaded, for controlling a Trimble SSeries instrument the
Trimble.Ssi.Driver.CarpoBased.Driver.SSeries.Windows.dll
must be loaded.
If the driver loaded successfully a _sensor
can be created - which is an instance of the driver.
IDriverManager driverManager = new DriverManager(IsvDetails.Developer);
try
{
var driverDir = Directory.GetCurrentDirectory() + @".\..\..\..\lib\ssi\" + @"Trimble.Ssi.Driver.CarpoBased.Driver.SSeries.Windows.dll";
Assembly driverAssembly = Assembly.LoadFrom(driverDir);
driverManager.RegisterDriverAssembly(driverAssembly);
IList<IDriverInformation> drivers = driverManager.ListAvailableDrivers();
IDriverInformation driverInformation = drivers.FirstOrDefault();
var driver = driverManager.CreateDriver(driverInformation);
_sensor = driver.CreateSensor();
}
catch (Exception e)
{
throw;
}
Connect
Now that we have a valid _sensor
we can try to connect to the sensor. The easiest way in case of an Trimble SSeries is via USB cable as there are no parameters required. More complex examples like Bluetooth connections are shown inside the documentation.
if (_sensor.IsSupported(ConnectionType.Usb))
{
var connectionSettingsContainer = new ConnectionSettings(ConnectionType.Usb);
try
{
await Task.Run(() => _sensor.Connect(connectionSettingsContainer));
Initialize();
return true;
}
catch (Exception e)
{
exceptionCallback(e);
}
}
Note that every function inside the SDK is provided in both sync and async manner. As the SDK supports also .NET version below 4.5 there is no async
and await
pattern but the more traditional Begin
and End
pattern (APM pattern).
The above approach wraps the sync function into a async Task which is easy to integrate in MVVM based applications (so basically UI centric apps).