C# facade integration
The following few steps explain how to integrated the TPSDK facade into an Visual Studio C# Solution. Note that the following steps are meant to guide the developer - as always there are multiple ways to achieve the same.
.NET MAUI vs Xamarin Forms
Starting with .NET6 Microsoft® introduced a new toolset to built cross-platform native mobile applications: .NET Multi-platform App UI (.NET MAUI).
This toolset replaced Xamarin® and Xamarin FormsTM.
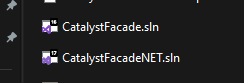
As a consequence TPSDK is provided in two flavors:
- the older Xamarin version
- the newer NET8 version
While the first one is meant for applications that are still in the transition period the second one is the go-to version for any new development.
Xamarin
Create a new Project
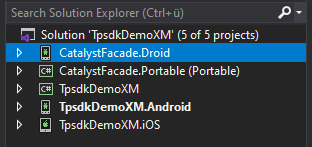
Create a new project in Visual Studio - typically this is a Mobile App (Xamarin.Forms)
or similar. The template or style of the app does not matter in this context.
Cross platform mobile apps have usually a platform specific project and a shared one.
Within TPSDK the shared code is within the portable
project while platform specific implementations are in the corresponding platform
projects.
Add projects and references
Adding the facade to your solution
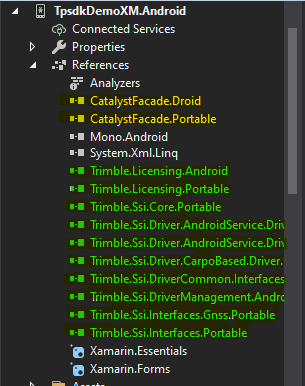
After the solution is created add the CatalystFacade.Portable
and CatalystFacade.Droid
projects to your solution.
The purpose of adding those two is that you can easily improve the facade interface and extend it to your needs. You can also just reference the CatalystFacade.Portable.dll
and CatalystFacade.Droid.dll
libraries.
After adding the projects you need to reference both in your main project. Therefore click on your platform project (TpsdkDemoXm.Android in this example) and either
- Select
Add
andReference
and selectProjects
and tick the check boxes for both facade projects - Select
Add
andReference
and browse to the two Facade dlls as part of the facade sample
Adding references to your solution
ABI files
After adding the facade and portable projects its necessary to add the remaining libraries as available in the libs
folder of TPSDK.
This work is unfortunately a bit cumbersome due to the multiple Android binary interfaces (ABI) but identical file names.
The most practical way to the best of our knowledge is:
- Copy all ABI folders from the SDK into a jniLibs folder in your project/ solution - create a similar folder setup as in [java](\ref GuideAndroidStudio) if not already available
- In the Solution Explorer of Visual Studio, add a filter called ‘jniLibs’ or ‘jni’ or similar
- Further on do a right mouse click on the folder created in 2 and select ‘add an existing item’. In the following dialog select all
so
files of a single ABI and add them as link. - Change the
Build Action
fromNone
toAndroidNativeLibrary
. If you’re building not an application but dll please review the [Microsoft documentation](\ref https://docs.microsoft.com/en-us/xamarin/android/platform/native-libraries) regarding the correct build action. - Close the solution and open the
csproj
file of your solution - Navigate to the single included
AndroidNativeLibrary
file and 6.1. Adjust all accordingly 6.2. Copy/ paste all so-file references and adjust the ABI
Its important to keep the structure of the libs
folder as the native files look explicitly for this structure.
In the end the
<ItemGroup>
<AndroidNativeLibrary Include=".\..\libs\arm64-v8a\libc++_shared.so" />
<AndroidNativeLibrary Include=".\..\libs\arm64-v8a\libDRV_TrimbleCommon.so" />
...
<AndroidNativeLibrary Include=".\..\libs\arm64-v8a\libTrimble.Ssi.Interfaces.GNSS.so" />
<AndroidNativeLibrary Include=".\..\libs\armeabi-v7a\libc++_shared.so" />
<AndroidNativeLibrary Include=".\..\libs\armeabi-v7a\libDRV_TrimbleCommon.so" />
<AndroidNativeLibrary Include=".\..\libs\armeabi-v7a\libDRV_TrimCom.so" />
...
<AndroidNativeLibrary Include=".\..\libs\armeabi-v7a\libTrimble.Ssi.Interfaces.Common.so" />
<AndroidNativeLibrary Include=".\..\libs\armeabi-v7a\libTrimble.Ssi.Interfaces.GNSS.so" />
...
</ItemGroup>
DLL references
In contrast to the ABI import this is rather straight forward:
All dll
s must get referenced via the Visual Studio reference importer.
Note that all ‘portable’ dlls must be imported to the portable project (if available); everything else goes into the Android specific project.
You may have noticed that the folder contains more dlls then mentioned above - for example Trimble.Ssi.Driver.MockGnss.Android.dll
.
These are mostly optional libraries or get loaded dynamically.
For example the already mentioned Trimble.Ssi.Driver.MockGnss.Android.dll
is a mock driver that simulates a receiver and can be used for testing purpose.
There are other optional libraries like Trimble.EMPOWER.*.dll
that handle most things related to Trimble EMPOWER modules.
In case you are missing a specific hardware feature its best to double check the content of the lib folder - if the hardware in question has a specific optional library.
Using the facade
At this stage we have a project which builds successfully and is ready to go. We can now initialize that facade via something like
CatalystFacade.Droid.CatalystFacade _catalystFacade = new CatalystFacade.Droid.CatalystFacade(myAppGUID, this);
where myAppGUID
is either the GUID from the facade sample or the received GUID from Trimble.
Note that the manifests permission need some adjustment before deploying the application. For details on manifest permission please check the documentation.